Dalam tutorial ini kita akan membahas tentang CRUD sederhana (Create, Read, Update, Delete) operasi PHP, ini adalah beberapa hal dasar dari aplikasi web PHP, Records adalah insert, select update dan menghapus menggunakan PHP dan MySQL, belajar operasi crud adalah cara pertama untuk memahami ini disini saya akan membuat tutorial sederhana dan mudah mari kita lihat cara pembuatannya.
Desain Database dan Tabel yang digunakan dalam tutorial ini.
Nama Database: dbtuts
Nama tabel: users
Tabel Kolom: first_name , last_name , user_city
Copy Paste-skema sql berikut dalam database MySql Anda untuk membuat database dan tabel.
konfigurasi database anda
dbconfig.php
Untuk menyediakan antarmuka pengguna untuk memasukkan database, kita harus membuat formulir, berisi semua bidang tabel pengguna memiliki, kecuali user_id yang otomatis dihasilkan bidang numerik. Dan, kode HTML bentuk Masukkan akan sebagai berikut.
add_data.php
Di atas tag html kita perlu menempatkan kode PHP yang menangani koneksi database dan memasukkan catatan baru ke dalam tabel pengguna, Jadi kode tersebut adalah sebagai berikut: menempatkan php kode insert berikut tepat di atas mulai tag.
Setelah memasukkan data kita perlu untuk mengambil semua catatan dari meja pengguna:
Mengambil Records Dari tabel pengguna Berikut adalah kode php untuk memilih catatan dari tabel.
index.php
Output :
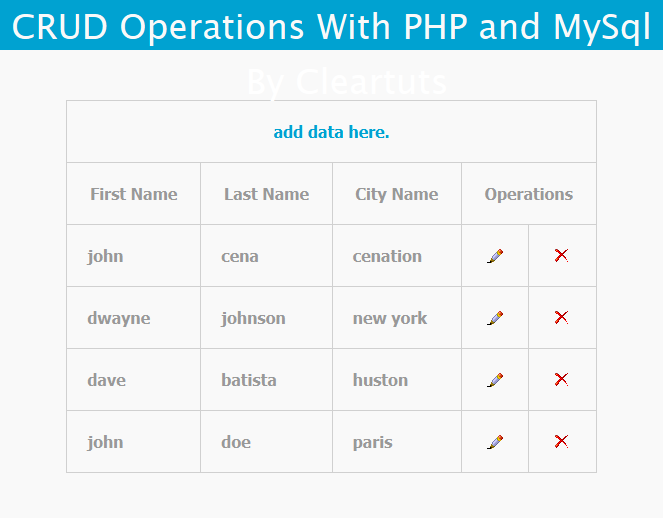
untuk menyelesaikan operasi ini dengan setiap record kita dapat menggunakan mengedit ikon yang menampilkan dengan setiap record seperti yang ditunjukkan di atas screenshot pada mengklik icon ini untuk baris tertentu halaman akan melompat ke mengedit data.php dengan baris tertentu dan rincian yang akan diedit yang diisi dalam formulir di bawah, dan itu akan terlihat seperti.
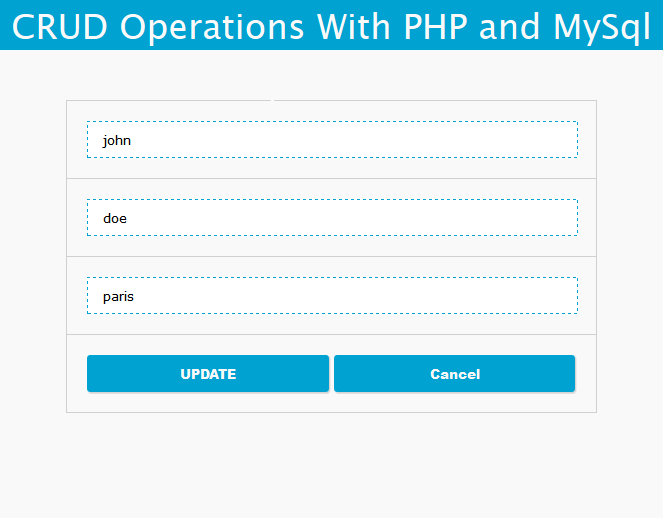
Untuk itu kita perlu untuk mengambil baris yang dipilih dari tabel pengguna dengan menggunakan SELECT Query dan QueryString yang diatur dalam mengedit url data.php dengan mengedit _id = nilai.
sekarang ada operasi CRUD selesai.
itu dia
dbconfig.php
style.css
Desain Database dan Tabel yang digunakan dalam tutorial ini.
Nama Database: dbtuts
Nama tabel: users
Tabel Kolom: first_name , last_name , user_city
Copy Paste-skema sql berikut dalam database MySql Anda untuk membuat database dan tabel.
CREATE DATABASE `dbtuts` ;
CREATE TABLE `dbtuts`.`users` (
`user_id` INT( 10 ) NOT NULL AUTO_INCREMENT PRIMARY KEY ,
`first_name` VARCHAR( 25 ) NOT NULL ,
`last_name` VARCHAR( 25 ) NOT NULL ,
`user_city` VARCHAR( 45 ) NOT NULL
) ENGINE = InnoDB;
style.csskonfigurasi database anda
dbconfig.php
<?php
$host = "localhost";
$user = "root";
$password = "";
$datbase = "dbtuts";
mysql_connect($host,$user,$password);
mysql_select_db($datbase);
?>
Buat : Insert New Records
Untuk menyediakan antarmuka pengguna untuk memasukkan database, kita harus membuat formulir, berisi semua bidang tabel pengguna memiliki, kecuali user_id yang otomatis dihasilkan bidang numerik. Dan, kode HTML bentuk Masukkan akan sebagai berikut.
add_data.php
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>CRUD Operations With PHP and MySql - By Cleartuts</title>
<link rel="stylesheet" href="style.css" type="text/css" />
</head>
<body>
<center>
<div id="header">
<div id="content">
<label>CRUD Operations With PHP and MySql - By Cleartuts</label>
</div>
</div>
<div id="body">
<div id="content">
<form method="post">
<table align="center">
<tr>
<td align="center"><a href="index.php">back to main page</a></td>
</tr>
<tr>
<td><input type="text" name="first_name" placeholder="First Name" required /></td>
</tr>
<tr>
<td><input type="text" name="last_name" placeholder="Last Name" required /></td>
</tr>
<tr>
<td><input type="text" name="city_name" placeholder="City" required /></td>
</tr>
<tr>
<td><button type="submit" name="btn-save"><strong>SAVE</strong></button></td>
</tr>
</table>
</form>
</div>
</div>
</center>
</body>
Di atas tag html kita perlu menempatkan kode PHP yang menangani koneksi database dan memasukkan catatan baru ke dalam tabel pengguna, Jadi kode tersebut adalah sebagai berikut: menempatkan php kode insert berikut tepat di atas mulai tag.
<?php
include_once 'dbconfig.php';
if(isset($_POST['btn-save']))
{
// variables for input data
$first_name = $_POST['first_name'];
$last_name = $_POST['last_name'];
$city_name = $_POST['city_name'];
// variables for input data
// sql query for inserting data into database
$sql_query = "INSERT INTO users(first_name,last_name,user_city) VALUES('$first_name','$last_name','$city_name')";
mysql_query($sql_query);
// sql query for inserting data into database
}
?>
Baca : Mengambil Records dari tabel
Setelah memasukkan data kita perlu untuk mengambil semua catatan dari meja pengguna:
Mengambil Records Dari tabel pengguna Berikut adalah kode php untuk memilih catatan dari tabel.
$sql_query="SELECT * FROM users";
$result_set=mysql_query($sql_query);
Menggunakan berikut ketika () loop kita dapat mengambil semua catatan dari meja pengguna. Sebagai contoh.<?php
$sql_query="SELECT * FROM users";
$result_set=mysql_query($sql_query);
while($row = mysql_fetch_array($result))
{
?>
<!--Add HTML code here to display records.-->
<?php
}
?>
Setelah itu, daftar catatan database akan ditampilkan ke browser, seperti yang ditunjukkan di bawah ini. Dan, daftar ini berisi ikon untuk memicu update dan menghapus operasi untuk setiap record.index.php
<?php
include_once 'dbconfig.php';
?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>CRUD Operations With PHP and MySql - By Cleartuts</title>
<link rel="stylesheet" href="style.css" type="text/css" />
</head>
<body>
<center>
<div id="body">
<div id="content">
<table align="center">
<tr>
<th colspan="5"><a href="add_data.php">add data here.</a></th>
</tr>
<th>First Name</th>
<th>Last Name</th>
<th>City Name</th>
<th colspan="2">Operations</th>
</tr>
<?php
$sql_query="SELECT * FROM users";
$result_set=mysql_query($sql_query);
while($row=mysql_fetch_row($result_set))
{
?>
<tr>
<td><?php echo $row[1]; ?></td>
<td><?php echo $row[2]; ?></td>
<td><?php echo $row[3]; ?></td>
<td align="center"><a href="javascript:edt_id('<?php echo $row[0]; ?>')"><img src="b_edit.png" align="EDIT" /></a></td>
<td align="center"><a href="javascript:delete_id('<?php echo $row[0]; ?>')"><img src="b_drop.png" align="DELETE" /></a></td>
</tr>
<?php
}
?>
</table>
</div>
</div>
</center>
</body>
</html>
Output :
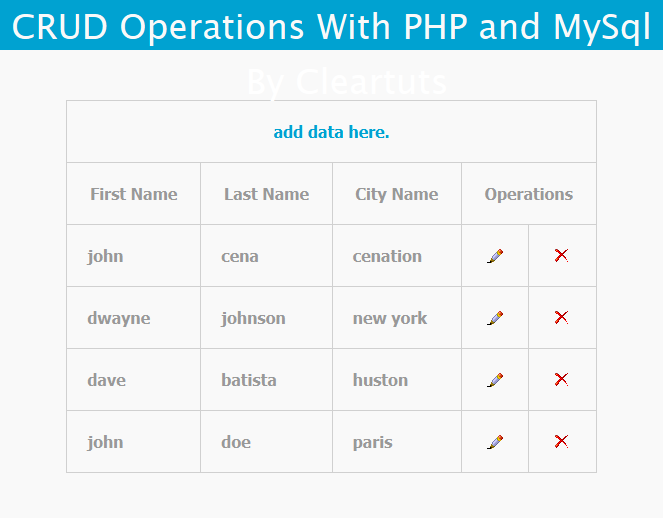
Update : Update MySQL Records.
untuk menyelesaikan operasi ini dengan setiap record kita dapat menggunakan mengedit ikon yang menampilkan dengan setiap record seperti yang ditunjukkan di atas screenshot pada mengklik icon ini untuk baris tertentu halaman akan melompat ke mengedit data.php dengan baris tertentu dan rincian yang akan diedit yang diisi dalam formulir di bawah, dan itu akan terlihat seperti.
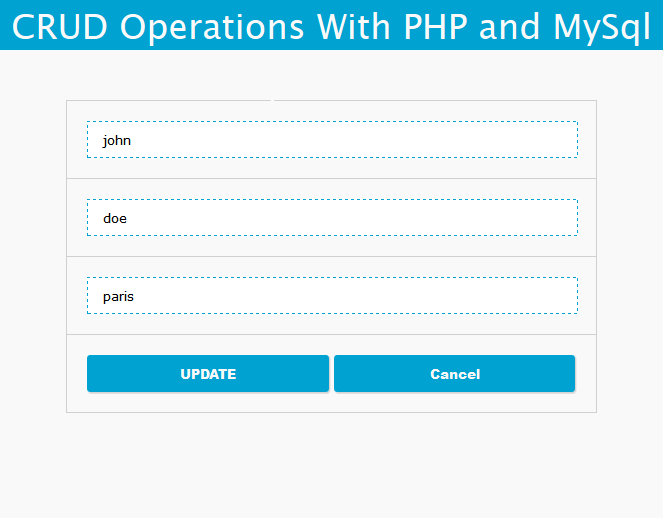
Untuk itu kita perlu untuk mengambil baris yang dipilih dari tabel pengguna dengan menggunakan SELECT Query dan QueryString yang diatur dalam mengedit url data.php dengan mengedit _id = nilai.
if(isset($_GET['edit_id']))
{
$sql_query="SELECT * FROM users WHERE user_id=".$_GET['edit_id'];
$result_set=mysql_query($sql_query);
$fetched_row=mysql_fetch_array($result_set);
}
setelah memilih data yang kita butuhkan untuk menjalankan UPDATE Query sebagai berikutif(isset($_POST['btn-update']))
{
// variables for input data
$first_name = $_POST['first_name'];
$last_name = $_POST['last_name'];
$city_name = $_POST['city_name'];
// variables for input data
// sql query for update data into database
$sql_query = "UPDATE users SET first_name='$first_name',last_name='$last_name',user_city='$city_name' WHERE user_id=".$_GET['edit_id'];
mysql_query($sql_query);
// sql query for update data into database
}
.edit_data.php <?php
include_once 'dbconfig.php';
if(isset($_GET['edit_id']))
{
$sql_query="SELECT * FROM users WHERE user_id=".$_GET['edit_id'];
$result_set=mysql_query($sql_query);
$fetched_row=mysql_fetch_array($result_set);
}
if(isset($_POST['btn-update']))
{
// variables for input data
$first_name = $_POST['first_name'];
$last_name = $_POST['last_name'];
$city_name = $_POST['city_name'];
// variables for input data
// sql query for update data into database
$sql_query = "UPDATE users SET first_name='$first_name',last_name='$last_name',user_city='$city_name' WHERE user_id=".$_GET['edit_id'];
mysql_query($sql_query));
// sql query for update data into database
}
?>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>CRUD Operations With PHP and MySql - By Cleartuts</title>
<link rel="stylesheet" href="style.css" type="text/css" />
</head>
<body>
<center>
<div id="body">
<div id="content">
<form method="post"><
<table align="center">
<tr>
<td><input type="text" name="first_name" placeholder="First Name" value="<?php echo $fetched_row['first_name']; ?>" required /></td>
</tr>
<tr>
<td><input type="text" name="last_name" placeholder="Last Name" value="<?php echo $fetched_row['last_name']; ?>" required /></td>
</tr>
<tr>
<td><input type="text" name="city_name" placeholder="City" value="<?php echo $fetched_row['user_city']; ?>" required /></td>
</tr>
<tr>
<td>
<button type="submit" name="btn-update"><strong>UPDATE</strong></button>
</td>
</tr>
</table>
</form>
</div>
</div>
</center>
</body>
</html>
Delete : Hapus Records dari tabel mysql.
Dengan mengklik ikon hapus dari catatan pengguna tertentu, pengguna id akan diatur ke URL QueryString halaman dan Query String index.php? Menghapus jika = nilai apapun jika mengatur Sql Query DELETE akan dieksekusi oleh if (isset ($ _GET ['delete_id'])) fungsi, dan menghapus pernyataan adalah sebagai berikut:if(isset($_GET['delete_id']))
{
$sql_query="DELETE FROM users WHERE user_id=".$_GET['delete_id'];
mysql_query($sql_query);
header("Location: $_SERVER[PHP_SELF]");
}
menempatkan di atas menghapus kode php dalam file index.php di atas data kode insert.sekarang ada operasi CRUD selesai.
itu dia
dbconfig.php
<?php
$host = "localhost";
$user = "root";
$password = "";
$datbase = "dbtuts";
mysql_connect($host,$user,$password);
mysql_select_db($datbase);
?>
index.php <?php
include_once 'dbconfig.php';
// delete condition
if(isset($_GET['delete_id']))
{
$sql_query="DELETE FROM users WHERE user_id=".$_GET['delete_id'];
mysql_query($sql_query);
header("Location: $_SERVER[PHP_SELF]");
}
// delete condition
?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>CRUD Operations With PHP and MySql - By Cleartuts</title>
<link rel="stylesheet" href="style.css" type="text/css" />
<script type="text/javascript">
function edt_id(id)
{
if(confirm('Sure to edit ?'))
{
window.location.href='edit_data.php?edit_id='+id;
}
}
function delete_id(id)
{
if(confirm('Sure to Delete ?'))
{
window.location.href='index.php?delete_id='+id;
}
}
</script>
</head>
<body>
<center>
<div id="header">
<div id="content">
<label>CRUD Operations With PHP and MySql - <a href="http://cleartuts.blogspot.com" target="_blank">By Cleartuts</a></label>
</div>
</div>
<div id="body">
<div id="content">
<table align="center">
<tr>
<th colspan="5"><a href="add_data.php">add data here.</a></th>
</tr>
<th>First Name</th>
<th>Last Name</th>
<th>City Name</th>
<th colspan="2">Operations</th>
</tr>
<?php
$sql_query="SELECT * FROM users";
$result_set=mysql_query($sql_query);
while($row=mysql_fetch_row($result_set))
{
?>
<tr>
<td><?php echo $row[1]; ?></td>
<td><?php echo $row[2]; ?></td>
<td><?php echo $row[3]; ?></td>
<td align="center"><a href="javascript:edt_id('<?php echo $row[0]; ?>')"><img src="b_edit.png" align="EDIT" /></a></td>
<td align="center"><a href="javascript:delete_id('<?php echo $row[0]; ?>')"><img src="b_drop.png" align="DELETE" /></a></td>
</tr>
<?php
}
?>
</table>
</div>
</div>
</center>
</body>
</html>
add_data.php <?php
include_once 'dbconfig.php';
if(isset($_POST['btn-save']))
{
// variables for input data
$first_name = $_POST['first_name'];
$last_name = $_POST['last_name'];
$city_name = $_POST['city_name'];
// variables for input data
// sql query for inserting data into database
$sql_query = "INSERT INTO users(first_name,last_name,user_city) VALUES('$first_name','$last_name','$city_name')";
// sql query for inserting data into database
// sql query execution function
if(mysql_query($sql_query))
{
?>
<script type="text/javascript">
alert('Data Are Inserted Successfully ');
window.location.href='index.php';
</script>
<?php
}
else
{
?>
<script type="text/javascript">
alert('error occured while inserting your data');
</script>
<?php
}
// sql query execution function
}
?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>CRUD Operations With PHP and MySql - By Cleartuts</title>
<link rel="stylesheet" href="style.css" type="text/css" />
</head>
<body>
<center>
<div id="header">
<div id="content">
<label>CRUD Operations With PHP and MySql - By Cleartuts</label>
</div>
</div>
<div id="body">
<div id="content">
<form method="post">
<table align="center">
<tr>
<td align="center"><a href="index.php">back to main page</a></td>
</tr>
<tr>
<td><input type="text" name="first_name" placeholder="First Name" required /></td>
</tr>
<tr>
<td><input type="text" name="last_name" placeholder="Last Name" required /></td>
</tr>
<tr>
<td><input type="text" name="city_name" placeholder="City" required /></td>
</tr>
<tr>
<td><button type="submit" name="btn-save"><strong>SAVE</strong></button></td>
</tr>
</table>
</form>
</div>
</div>
</center>
</body>
</html>
edit_data.php <?php
include_once 'dbconfig.php';
if(isset($_GET['edit_id']))
{
$sql_query="SELECT * FROM users WHERE user_id=".$_GET['edit_id'];
$result_set=mysql_query($sql_query);
$fetched_row=mysql_fetch_array($result_set);
}
if(isset($_POST['btn-update']))
{
// variables for input data
$first_name = $_POST['first_name'];
$last_name = $_POST['last_name'];
$city_name = $_POST['city_name'];
// variables for input data
// sql query for update data into database
$sql_query = "UPDATE users SET first_name='$first_name',last_name='$last_name',user_city='$city_name' WHERE user_id=".$_GET['edit_id'];
// sql query for update data into database
// sql query execution function
if(mysql_query($sql_query))
{
?>
<script type
style.css
@charset "utf-8";
/* CSS Document */
*
{
margin:0;
padding:0;
}
body
{
background:#f9f9f9;
font-family:"Courier New", Courier, monospace;
}
#header
{
width:100%;
height:50px;
background:#00a2d1;
color:#f9f9f9;
font-family:"Lucida Sans Unicode", "Lucida Grande", sans-serif;
font-size:35px;
text-align:center;
}
#header a
{
color:#fff;
text-decoration:blink;
}
#body
{
margin-top:50px;
}
table
{
width:80%;
font-family:Tahoma, Geneva, sans-serif;
font-weight:bolder;
color:#999;
margin-bottom:80px;
}
table a
{
text-decoration:none;
color:#00a2d1;
}
table,td,th
{
border-collapse:collapse;
border:solid #d0d0d0 1px;
padding:20px;
}
table td input
{
width:97%;
height:35px;
border:dashed #00a2d1 1px;
padding-left:15px;
font-family:Verdana, Geneva, sans-serif;
box-shadow:0px 0px 0px rgba(1,0,0,0.2);
outline:none;
}
table td input:focus
{
box-shadow:inset 1px 1px 1px rgba(1,0,0,0.2);
outline:none;
}
table td button
{
border:solid #f9f9f9 0px;
box-shadow:1px 1px 1px rgba(1,0,0,0.2);
outline:none;
background:#00a2d1;
padding:9px 15px 9px 15px;
color:#f9f9f9;
font-family:Arial, Helvetica, sans-serif;
font-weight:bolder;
border-radius:3px;
width:49.5%;
}
table td button:active
{
position:relative;
top:1px;
}
Cara Membuat CRUD (Create, Read, Update, Delete) PHP MySQL
4/
5
Oleh
Unknown
3 comments
Tulis commentsHai kak. Terimakasih ya kak artikel nya bagus mudah dipahami bagi saya yang pemula. Artikel nya juga sangat membantu dalan memenuhi tugas kuliah saya kak. Semoga dapat memberikan artikel yang bermanfaat lainnya ya kak. Terimakasih kak sukses selalu . Perkenalkan Saya Ardila Yunita, NIM 1922500096, link kampus https://www.atmaluhur.ac.id/
ReplyThanks kak, informasinya bermanfaat dan sangat membantu saya yang masih pemula ini.. Penjelasannya lengkap kak.. saya harap website kakak terus berkembang dengan karya" tulisan kakak, sukses slalu kak.. btw, saya Meren Laurensia mahasiswi dari ISB Atma Luhur Pangkalpinang.. skuy mampir ke website kampus saya kak, https://www.atmaluhur.ac.id/
ReplyTerima kak atas ilmu nya. Smga semakin jaya terus dan bisa bagi ilmu lagi.
ReplyJEFFRY H SUFRYANTO SIMARMATA
192250047
SI2J